WooCommerce offers a robust platform for building and managing online stores, and one of its powerful features is product variations. Variations allow you to offer different versions of a product, such as varying sizes, colors, or other attributes. In this blog post, we’ll explore how to extend WooCommerce variations by adding custom information specific to each variation.
Here’s what we’re building, see the “Diameter” and “Thichkness” displayed just above the Add to cart button.
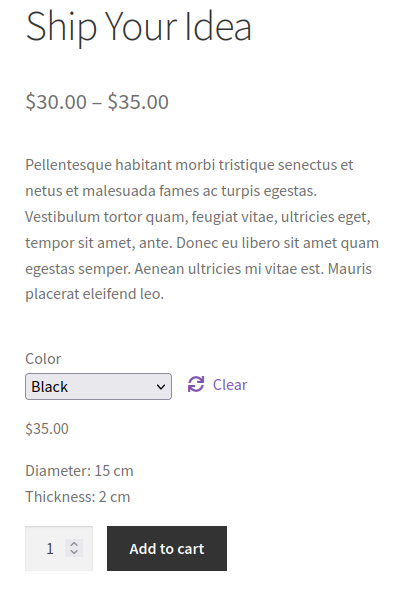
Custom Fields for Product Variation Settings
First, we need to add our custom field to the product variation settings. This allows you to include additional data for each variation in the WooCommerce admin. We are going to add some fields for thickness
and diameter
.
/**
* Add custom fields to product variation settings
*
* @param string $loop
* @param array $variation_data
* @param WP_Post $variation
* @return array
*/
function kia_add_variation_options_other_dimensions( $loop, $variation_data, $variation ) {
$variation_obj = wc_get_product( $variation->ID );
$unit = get_option( 'woocommerce_dimension_unit' );
if ( class_exists( 'Automattic\WooCommerce\Utilities\I18nUtil' ) ) {
$unit = Automattic\WooCommerce\Utilities\I18nUtil::get_dimensions_unit_label( $unit );
}
woocommerce_wp_text_input( array(
'id' => 'diameter[' . $loop . ']',
'class' => 'short',
'label' => sprintf( esc_html__( 'Diameter (%s)', 'extra-variation-data' ), esc_html( $unit ) ),
'desc_tip' => 'true',
'description' => __( 'Some dimension data to display for this variation.', 'extra-variation-data' ),
'value' => $variation_obj->get_meta( '_diameter', true ),
'placeholder' => wc_format_localized_decimal( 0 ),
'wrapper_class' => 'form-row form-row-first',
) );
woocommerce_wp_text_input( array(
'id' => 'thickness[' . $loop . ']',
'class' => 'short',
'label' => sprintf( esc_html__( 'Thickness (%s)', 'extra-variation-data' ), esc_html( $unit ) ),
'desc_tip' => 'true',
'description' => __( 'Some dimension data to display for this variation.', 'extra-variation-data' ),
'value' => $variation_obj->get_meta( '_thickness', true ),
'placeholder' => wc_format_localized_decimal( 0 ),
'wrapper_class' => 'form-row form-row-last',
) );
}
add_action( 'woocommerce_product_after_variable_attributes', 'kia_add_variation_options_other_dimensions', 10, 3 );
Code language: PHP (php)
This code adds a “Test Data” field to the product variation settings, allowing you to input additional information for each variation.
Saving Custom Fields for Product Variations
Now we need to handle saving the custom field values entered in the WooCommerce admin.
/**
* Save product variation custom fields values
*
* @param WC_Product_Variation $variation The product variation object.
* @param int $i The iteration in the loop.
*/
function kia_save_variation_options_other_dimensions( $variation, $i ) {
if ( isset( $_POST['diameter'][$i] ) ) {
$variation->update_meta_data( '_diameter', wc_clean( $_POST['diameter'][$i] ) );
}
if ( isset( $_POST['thickness'][$i] ) ) {
$variation->update_meta_data( '_thickness', wc_clean( $_POST['thickness'][$i] ) );
}
}
add_action( 'woocommerce_admin_process_variation_object', 'kia_save_variation_options_other_dimensions', 10, 2 );
Code language: PHP (php)
This code ensures that the custom field values (in this case, ‘diameter’ and ‘thickness’) are saved when changes are made in the WooCommerce admin.
Displaying Custom Data in the Frontend
The third snippet focuses on displaying the custom data in the frontend when a user selects a variation.
/**
* Add data to json encoded variation form
*
* @param array $data - This will be the variation's json data.
* @param WC_Product_Variable $product
* @param WC_Product_Variation $variation
* @return array
*/
function kia_available_variation( $data, $product, $variation ) {
$diameter = $variation->get_meta( '_diameter', true );
$new_data['diameter_html'] = $diameter ? sprintf( esc_html__( 'Diameter: %s', 'extra-variation-data' ), kia_format_single_dimensions( $diameter ) ) : '';
$thickness = $variation->get_meta( '_thickness', true );
$new_data['thickness_html'] = $thickness ? sprintf( esc_html__( 'Thickness: %s', 'extra-variation-data' ), kia_format_single_dimensions( $thickness ) ) : '';
return array_merge( $data, $new_data );
}
add_filter( 'woocommerce_available_variation', 'kia_available_variation', 10, 3 );
Code language: PHP (php)
This code adds the custom data to the JSON encoded variation form, ensuring it is available for use in the frontend.
Since WooCommerce doesn’t have a function for formatting a single dimension (only an array of dimensions relative to the Weight x Length x Height) we’ll create a little helper utility for ourselves:
/**
* Utility to format dimension for display.
*
* @param string $dimension_string Single dimension.
* @return string
*/
function kia_format_single_dimensions( $dimension_string ) {
if ( ! empty( $dimension_string ) ) {
$dimension_label = get_option( 'woocommerce_dimension_unit' );
if ( class_exists( 'Automattic\WooCommerce\Utilities\I18nUtil' ) ) {
$dimension_label = Automattic\WooCommerce\Utilities\I18nUtil::get_dimensions_unit_label( $dimension_label );
}
$dimension_string = sprintf(
// translators: 1. A formatted number; 2. A label for a dimensions unit of measure. E.g. 3.14 cm.
esc_html_x( '%1$s %2$s', 'formatted dimensions', 'extra-variation-data' ),
$dimension_string,
$dimension_label
);
} else {
$dimension_string = esc_html__( 'N/A', 'extra-variation-data' );
}
return $dimension_string;
}
Code language: PHP (php)
This should take the dimension and return the dimension formatted in the store units, example: 30 cm.
Adding a <div> to Hold the Ouput on the front end
It’s not possible to add fields into the single-product/add-to-cart/variation.php
template directly. Well, not without overriding that template, which is an option. But instead we will insert a little div that will can then use to render our custom fields on the front end.
/**
* Add a <div> element to hold dimension output.
* The class name needs to match the find() statement in kia_variable_footer_scripts()
*/
function kia_add_variation_dimension_holder() {
echo '<div class="woocommerce-variation woocommerce-variation-dimension-data"></div>';
}
add_action( 'woocommerce_single_variation', 'kia_add_variation_dimension_holder', 15 );
Code language: PHP (php)
Displaying on the Front End with JavaScript
The final snippet enhances the user experience by dynamically displaying the custom data when a variation is selected. WooCommerce uses a little backbone template to render it’s variation data when the variation is selected (at least until the product blocks change everything) and so we will use the same.
/**
* Add scripts to variable products.
*/
function kia_on_found_template_for_variable_add_to_cart() {
add_action( 'wp_print_footer_scripts', 'kia_variable_footer_scripts', 99 );
}
add_action( 'woocommerce_variable_add_to_cart', 'kia_on_found_template_for_variable_add_to_cart', 30 );
/**
* Print backbone template and inline scripts in the footer.
*/
function kia_variable_footer_scripts() { ?>
<script type="text/template" id="tmpl-variation-template-dimension-data"></script>
<script type="text/javascript">
jQuery( document ).ready(function($) {
$('form.cart')
.on('found_variation', function( event, variation ) {
template = wp.template( 'variation-template-dimension-data' );
$template_html = template( {
variation: variation
} );
$(this).find('.woocommerce-variation-dimension-data').html( $template_html ).slideDown();
})
.on( 'reset_data', function( event, variation ) {
$(this).find('.woocommerce-variation-dimension-data').slideUp( 200 );
});
});
</script>
<?php
}
Code language: JavaScript (javascript)
Full code can can be downloaded here.